These are all good answers, and yet there is another way: (Isn't there always?)
Due to the growing popularity of virtual reality, the folks at OculusVR have developed a trio of "Multiview" extensions called:
- OVR_multiview,
- OVR_multiview2, &
- OVR_multiview_multisampled_render_to_texture
These extensions allow multiple views of the same scene to be rendered in a single draw call, eliminating the redundancy of rendering the same scene in the same state from the viewpoint of each eye. Although created for the purpose of VR, the developer is not necessarily limited to merely two views. Rather, this extension allows for as many views as MAX_VIEWS_OVR specifies.
Before using these extensions, the developer should check for their support in the user's graphics driver by adding the following code:
const GLubyte* extensions = GL_CHECK( glGetString( GL_EXTENSIONS ) );
char * found_extension = strstr( (const char*)extensions, "GL_OVR_multiview" );
if (NULL == found_extension)
{
exit( EXIT_FAILURE );
}
From there, it is a matter of setting up your framebuffer to utilize this feature:
glFramebufferTextureMultisampledMultiviewOVR = PFNGLFRAMEBUFFERTEXTUREMULTISAMPLEDMULTIVIEWOVR(eglGetProcAddress("glFramebufferTextureMultisampleMultiviewOVR"));
glFramebufferTextureMultisampledMultiviewOVR (GL_DRAW_FRAMEBUFFER, GL_COLOR_ATTACHMENT0, textureID, 0, 0, 2);
and likewise in the shader:
#version 300 es
#extension GL_OVR_multiview : enable
layout(num_views = 2) in;
in vec3 vertexPosition;
uniform mat4 MVP[2];
void main(){
gl_Position = MVP[gl_ViewID_OVR] * vec4(vertexPosition, 1.0f);
}
In a CPU-bound application, this extension can dramatically reduce rendering time, especially with more complex scenes:
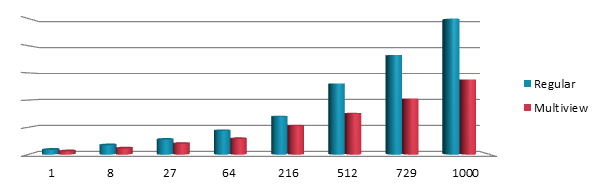