While creating a set of glTF models for a tutorial, I also intended to create THE minimal glTF file.
Update: The following referred to glTF 1.0/1.1. See below for an update of this example to glTF 2.0.
As already mentioned in the answer by 5chdn, one issue may be the material. According to the Appendix A: Default Material of the specification, an asset that does not contain a material should be rendered with a default material that simply shows the object in a uniform, 50% gray color.
Some viewers might not support this, and even if they do, there have been some minor subtleties in the spec regarding this point: In glTF 1.0, it was still necessary to define an "empty" material, because the mesh.primitive.material
property was not yet optional. But this should be fixed in glTF 1.1.
So the following should indeed be THE minimal possible glTF asset (version 1.1) :
{
"scenes" : {
"scene0" : {
"nodes" : [ "node0" ]
}
},
"nodes" : {
"node0" : {
"meshes" : [ "mesh0" ]
}
},
"meshes" : {
"mesh0" : {
"primitives" : [ {
"attributes" : {
"POSITION" : "positionsAccessor"
}
} ]
}
},
"buffers" : {
"buffer0" : {
"uri" : "data:application/octet-stream;base64,AAAAAAAAAAAAAAAAAACAPwAAAAAAAAAAAAAAAAAAgD8AAAAA",
"byteLength" : 36
}
},
"bufferViews" : {
"positionsBufferView" : {
"buffer" : "buffer0",
"byteOffset" : 0,
"byteLength" : 36,
"target" : 34962
}
},
"accessors" : {
"positionsAccessor" : {
"bufferView" : "positionsBufferView",
"byteOffset" : 0,
"componentType" : 5126,
"count" : 3,
"type" : "VEC3",
"max" : [ 1.0, 1.0, 0.0 ],
"min" : [ 0.0, 0.0, 0.0 ]
}
},
"asset" : {
"version" : "1.1"
}
}
It also shows a single triangle:
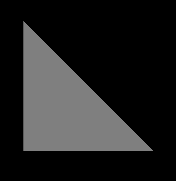
The version without embedded buffers is available at https://github.com/javagl/gltfTutorialModels , as well as a triangle with indices, at https://github.com/javagl/gltfTutorialModels/tree/master/Triangle
Update for glTF 2.0:
The concepts and top-level elements of glTF 2.0 are basically the same as for glTF 1.0. But there still have been some changes in the format. Most importantly (for this small example), the top-level dictionaries are now arrays. The following is the same "Triangle" model as above, updated accordingly:
{
"scenes":[
{
"nodes":[ 0 ]
}
],
"nodes":[
{
"mesh":0
}
],
"meshes":[
{
"primitives":[
{
"attributes":{
"POSITION":1
},
"indices":0
}
]
}
],
"buffers":[
{
"uri":"data:application/octet-stream;base64,AAABAAIAAAAAAAAAAAAAAAAAAAAAAIA/AAAAAAAAAAAAAAAAAACAPwAAAAA=",
"byteLength":44
}
],
"bufferViews":[
{
"buffer":0,
"byteOffset":0,
"byteLength":6,
"target":34963
},
{
"buffer":0,
"byteOffset":8,
"byteLength":36,
"target":34962
}
],
"accessors":[
{
"bufferView":0,
"byteOffset":0,
"componentType":5123,
"count":3,
"type":"SCALAR",
"max":[
2
],
"min":[
0
]
},
{
"bufferView":1,
"byteOffset":0,
"componentType":5126,
"count":3,
"type":"VEC3",
"max":[
1.0,
1.0,
0.0
],
"min":[
0.0,
0.0,
0.0
]
}
],
"asset":{
"version":"2.0"
}
}
This and other glTF sample models can be found in the glTF sample models repository.